To develop efficient programs, asynchronous programming is essential, and modern programming languages all support it.
Understanding Asynchrony
What exactly is asynchronous programming?
The opposite concept is synchronous programming, where tasks are completed one after another in sequential order. Asynchronous programming operates differently – multiple tasks can be executed in parallel or interleaved. Let’s use a real-life analogy to illustrate the difference.
Imagine preparing dinner tonight. Following a recipe:
- Pour oil into the pan
- Wait for it to heat
- Add ingredients and seasonings
- Stir-fry
- Plate and serve
Now you can eat while watching videos or listening to music – enjoying food and entertainment simultaneously.
In this analogy:
- Cooking is synchronous – each step must complete before the next begins
- Eating is asynchronous – you can alternate between rice and dishes while parallelizing dining with entertainment
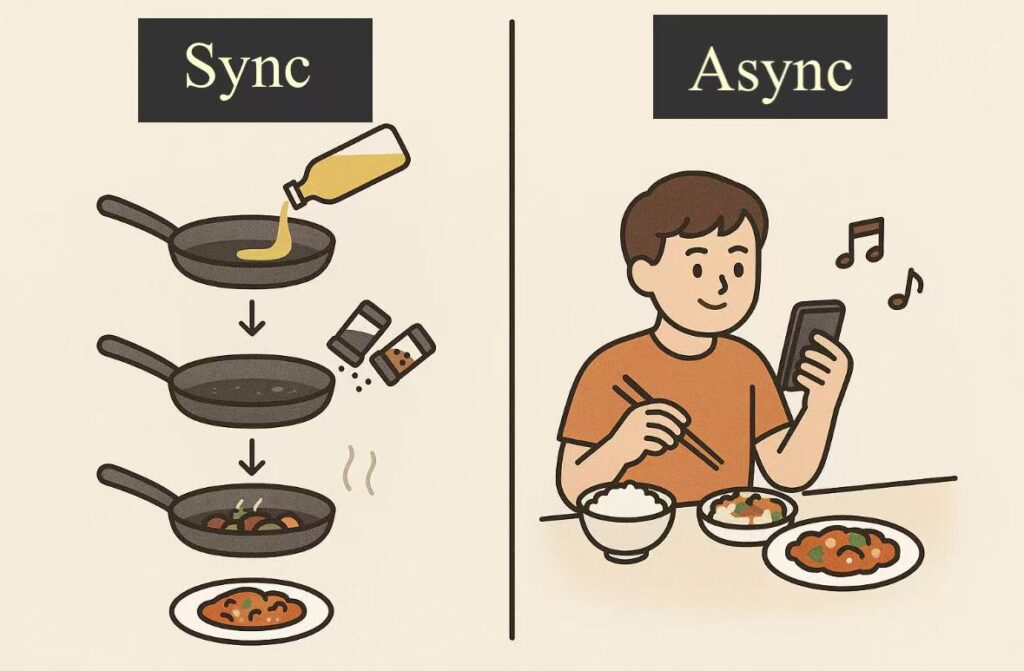
Now let’s examine how different programming languages implement asynchronous programming, focusing on Java, Golang, and Rust.
Java’s Approach
Java traditionally used multi-threading for asynchronous programming. The main thread spawns worker threads that execute independently:
public class AsyncDiner {
public static void main(String[] args) {
Thread eatRiceThread = new Thread(() -> {
try {
for (int i = 1; i <= 3; i++) {
System.out.println("Eating rice");
Thread.sleep(1000);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
// Additional thread definitions...
eatRiceThread.start();
// Start other threads...
try {
eatRiceThread.join();
// Join other threads...
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Modern Java offers additional approaches like CompletableFuture and third-party solutions for more efficient async programming.
Golang’s Goroutines
Golang implements asynchrony through goroutines – lightweight threads managed by Go’s runtime. Simply prefix function calls with go
:
package main
import (
"fmt"
"sync"
"time"
)
func eatRice(wg *sync.WaitGroup) {
defer wg.Done()
for i := 1; i <= 3; i++ {
fmt.Println("Eating rice")
time.Sleep(1 * time.Second)
}
}
func main() {
var wg sync.WaitGroup
wg.Add(3)
go eatRice(&wg)
// Launch other goroutines...
wg.Wait()
}
Rust’s Async/Await
Rust uses async/await syntax with community-provided runtimes like Tokio:
use tokio::time::{sleep, Duration};
async fn eat_rice() {
for _ in 1..=3 {
println!("Eating rice");
sleep(Duration::from_secs(1)).await;
}
}
#[tokio::main]
async fn main() {
tokio::join!(
eat_rice(),
// Other async tasks...
);
}
Requires Cargo.toml
dependency:
[dependencies]
tokio = { version = "1.45.0", features = ["full"] }
Threads vs. Coroutines
Key differences:
- Threads: OS-managed, preemptive scheduling, communicate via shared memory
- Coroutines: User-space lightweight threads, cooperative scheduling, communicate via channels
Threads execute on CPU cores with context-switching overhead. Coroutines run within threads, enabling many concurrent tasks with minimal switching cost.
Conclusion
We’ve explored synchronous vs. asynchronous concepts through cooking/eating analogies and demonstrated implementations in Java (threads), Golang (goroutines), and Rust (async/await). Each approach has optimal use cases:
- Threads: CPU-intensive tasks leveraging multiple cores (e.g., data processing)
- Coroutines: IO-bound tasks requiring high concurrency (e.g., web servers)
Future articles will explore Rust’s async internals and practical applications where async shines.